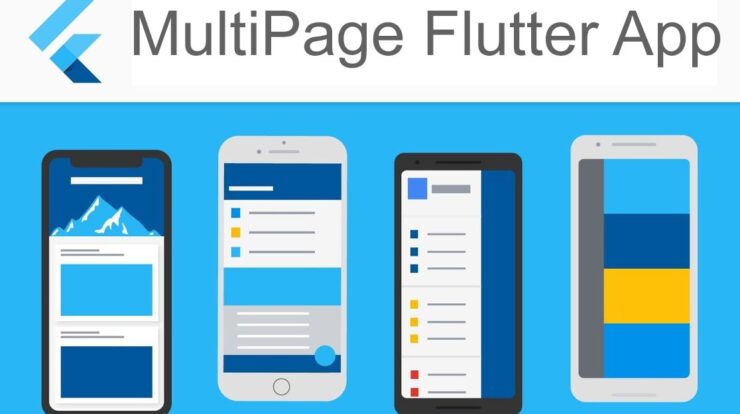
In this tutorial we gonna learn to create a very simple two page application using Flutter. We will be having the first page with a button. When the use clicks the button, navigation will happen to page two.
This is the easy way and useful method only for simple application. This approach is not suitable for complex applications.
We will be also passing some data from the first page. This data will be displayed in the page two.
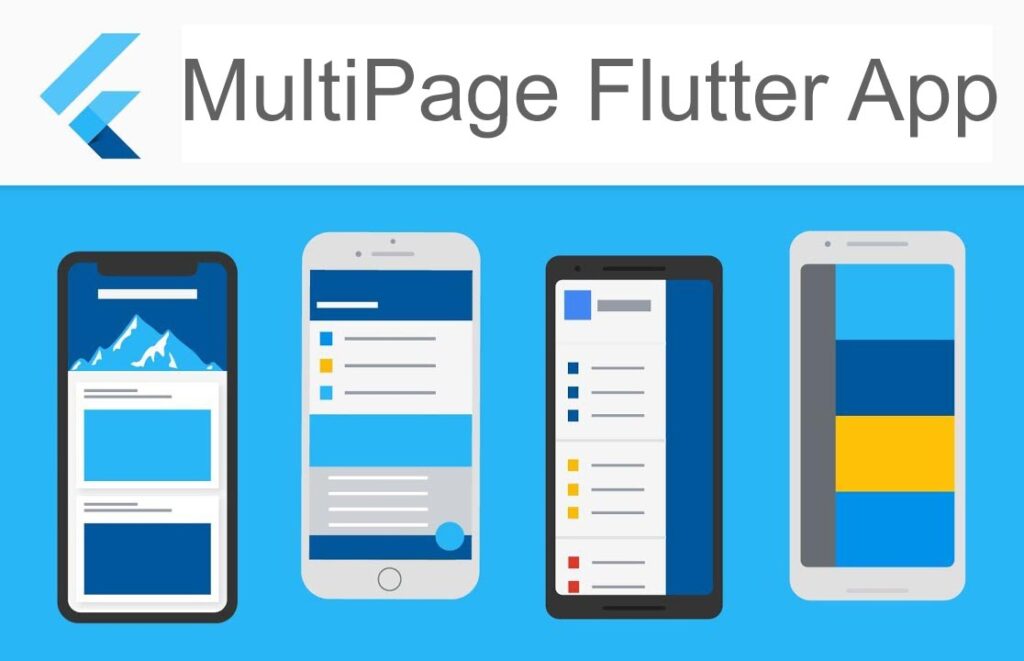
Lets Build the Simple Two Page Flutter App
- Firstly create a new app using the terminal code: flutter create multipage_app
You need to type the code in your terminal. Now the new multipage_app flutter project will be created. Sample code app can be seen.
- Now Edit the main.dart file in the lib folder
- Delete all the default code in the file and copy paste the below code..
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: FirstPage(),
);
}
}
class FirstPage extends StatelessWidget{
Widget build(BuildContext context){
return Scaffold(
appBar: AppBar(
title: Text('Routing App'),
),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(
'First Page',
style: TextStyle(fontSize: 50),
),
RaisedButton(
child: Text('Go to second'),
onPressed: (){
Navigator.of(context).push(
MaterialPageRoute(
builder: (context) => SecondPage(data: "Hello there from the first page!"),
),
);
})
]
)
),
);
}
}
class SecondPage extends StatelessWidget{
final String data;
SecondPage({
Key key,
@required this.data,
}): super(key:key);
Widget build(BuildContext context){
return Scaffold(
appBar: AppBar(
title: Text('Routing App'),
),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(
'Second Page',
style: TextStyle(fontSize: 50),
),
Text(
data,
style: TextStyle(fontSize: 20),
)
]
)
),
);
}
}
Tags: Flutter navigation, flutter multi-page app code, navigation from page 1 to page 2 in flutter , how to route pages in flutter – dart flutter simple apple codes